Synopsis Of Inheritance: A Comprehensive Guide To Understanding And Applying It
Inheritance is a cornerstone of object-oriented programming (OOP), offering a mechanism for reusability, scalability, and efficient code management. It allows one class to inherit properties and behaviors from another, fostering a hierarchical structure that mirrors real-world relationships. Whether you're a beginner exploring the basics or a seasoned developer looking to refine your understanding, inheritance plays a vital role in software development. By enabling code reuse and promoting modularity, inheritance not only simplifies programming but also enhances the maintainability of complex systems. Its applications span various domains, from web development to artificial intelligence, making it an indispensable tool in modern programming.
At its core, inheritance revolves around the concept of a "parent-child" relationship. A parent class, also known as a base or superclass, defines common attributes and methods that can be inherited by one or more child classes, or subclasses. This relationship not only reduces redundancy but also allows developers to build upon existing code, introducing new functionalities without rewriting the entire structure. For instance, a "Vehicle" class might define properties like "color" and "speed," while subclasses like "Car" and "Bike" inherit these attributes and add their unique features. This hierarchical organization is intuitive and mirrors how we categorize objects in the real world.
Understanding the synopsis of inheritance is essential for anyone working with OOP languages such as Java, Python, or C++. It provides a framework for designing robust and scalable applications, ensuring that code remains clean, efficient, and easy to maintain. In this article, we’ll delve into the intricacies of inheritance, exploring its types, benefits, and practical applications. Whether you're curious about how inheritance works or seeking answers to common questions, this guide will equip you with the knowledge to harness its full potential.
Read also:Young Celebs Who Died Tragic Stories That Shaped The Entertainment Industry
Table of Contents
- What is Inheritance and Why is it Important?
- What Are the Different Types of Inheritance?
- How Does Inheritance Work in Programming Languages?
- What Are the Key Benefits of Using Inheritance?
- Common Misconceptions About Inheritance
- Real-World Applications of Inheritance
- What Are the Challenges and Limitations of Inheritance?
- Frequently Asked Questions About Synopsis of Inheritance
What is Inheritance and Why is it Important?
Inheritance is a fundamental concept in object-oriented programming (OOP) that enables one class to inherit attributes and methods from another. This relationship is often described using the terms "parent" and "child," where the parent class (or superclass) serves as the blueprint, and the child class (or subclass) extends or modifies its functionality. The primary goal of inheritance is to promote code reuse, reduce redundancy, and create a logical hierarchy that reflects real-world relationships.
Why is inheritance so crucial in programming? For starters, it simplifies the development process by allowing developers to build upon existing code. Instead of writing the same methods and properties repeatedly, you can define them once in a parent class and reuse them across multiple subclasses. For example, in a banking application, a "BankAccount" class might define common attributes like "accountNumber" and "balance." Subclasses like "SavingsAccount" and "CheckingAccount" can inherit these attributes while adding their unique features, such as interest rates or overdraft limits.
Moreover, inheritance enhances the maintainability of code. When changes are needed, you can update the parent class, and the modifications will automatically propagate to all subclasses. This ensures consistency and reduces the risk of errors. Additionally, inheritance supports polymorphism, another key OOP principle, enabling objects of different classes to be treated as instances of a common superclass. This flexibility is invaluable in designing scalable and adaptable software systems.
How Does Inheritance Foster Reusability?
Reusability is one of the most significant advantages of inheritance. By allowing subclasses to inherit properties and methods from a parent class, developers can avoid duplicating code. This not only saves time but also minimizes the chances of introducing bugs. For instance, if you're developing a game, you might create a "Character" class with attributes like "health" and "speed." Subclasses like "Hero" and "Villain" can inherit these attributes while introducing their unique behaviors, such as special abilities or attack patterns.
Another way inheritance fosters reusability is through method overriding. Subclasses can redefine methods inherited from the parent class to suit their specific needs. For example, a "Shape" class might define a method called "calculateArea," while subclasses like "Circle" and "Rectangle" override this method to provide their own implementations. This approach ensures that the code remains modular and extensible.
Why Should Developers Prioritize Inheritance?
Prioritizing inheritance in software design offers several benefits. First, it promotes a clear and organized structure, making the code easier to understand and maintain. Second, it reduces development time by enabling developers to reuse existing code. Finally, it supports the creation of scalable systems that can adapt to changing requirements. By mastering inheritance, developers can build robust applications that are both efficient and flexible.
Read also:Khloe Kardashian Leggings The Ultimate Guide To Style Comfort And Quality
What Are the Different Types of Inheritance?
Inheritance can take several forms, each serving a specific purpose in software design. Understanding these types is crucial for leveraging inheritance effectively. Below, we explore the most common types of inheritance, along with examples to illustrate their applications.
Single Inheritance
Single inheritance occurs when a subclass inherits from a single parent class. This is the simplest form of inheritance and is widely used in programming. For example, consider a "Vehicle" class with attributes like "color" and "speed." A "Car" class can inherit these attributes while adding its own, such as "numberOfDoors." Single inheritance is straightforward and easy to implement, making it a popular choice for many applications.
Multiple Inheritance
Multiple inheritance allows a subclass to inherit from more than one parent class. While powerful, this type of inheritance can lead to complexities, such as the "diamond problem," where ambiguity arises if two parent classes define the same method. Languages like Python support multiple inheritance, while others, like Java, avoid it to prevent such issues. For example, a "FlyingCar" class might inherit from both "Car" and "Aeroplane" classes, combining their functionalities.
Multilevel Inheritance
Multilevel inheritance involves a chain of inheritance, where a subclass inherits from a parent class, which in turn inherits from another parent class. For instance, a "Grandparent" class might define basic attributes, a "Parent" class can extend these, and a "Child" class can further refine them. This type of inheritance is useful for creating deeply hierarchical structures but should be used cautiously to avoid excessive complexity.
Other Types of Inheritance
- Hierarchical Inheritance: Occurs when multiple subclasses inherit from a single parent class. For example, "Manager" and "Employee" classes might inherit from a common "Person" class.
- Hybrid Inheritance: Combines two or more types of inheritance, such as single and multiple inheritance, to create complex relationships.
How Does Inheritance Work in Programming Languages?
The implementation of inheritance varies across programming languages, but the underlying principles remain consistent. Understanding how inheritance works in different languages is essential for developers aiming to build cross-platform applications. Below, we explore how inheritance is implemented in some of the most popular programming languages.
Inheritance in Java
Java uses the "extends" keyword to establish inheritance between classes. A subclass can inherit fields and methods from a parent class, and it can also override methods to provide specific implementations. Java does not support multiple inheritance directly but achieves similar functionality using interfaces. For example:
class Animal { void eat() { System.out.println("This animal eats food."); } } class Dog extends Animal { void bark() { System.out.println("The dog barks."); } }
Inheritance in Python
Python supports multiple inheritance, making it a versatile choice for developers. The syntax is straightforward, using parentheses to specify the parent class. Python also allows method overriding and the use of the "super()" function to call methods from the parent class. For example:
class Animal: def speak(self): print("Animal speaks") class Dog(Animal): def speak(self): print("Dog barks")
Key Differences Across Languages
While the concept of inheritance is universal, its implementation varies. For instance, C++ supports multiple inheritance but requires careful handling to avoid ambiguity. On the other hand, JavaScript uses prototype-based inheritance, which differs significantly from class-based inheritance in languages like Java and Python. Understanding these nuances is crucial for writing efficient and error-free code.
What Are the Key Benefits of Using Inheritance?
Inheritance offers numerous advantages that make it an indispensable tool in software development. Below, we explore the key benefits of using inheritance and how they contribute to building efficient and maintainable applications.
Code Reusability and Efficiency
One of the primary benefits of inheritance is code reusability. By inheriting properties and methods from a parent class, developers can avoid duplicating code. This not only saves time but also reduces the likelihood of introducing bugs. For example, a "Shape" class might define a method for calculating area, which can be reused by subclasses like "Circle" and "Rectangle."
Scalability and Maintainability
Inheritance enhances the scalability of applications by enabling developers to extend existing functionality without modifying the original code. This is particularly useful in large projects where maintaining consistency is critical. Additionally, changes made to the parent class automatically propagate to all subclasses, ensuring that updates are applied uniformly.
Support for Polymorphism
Inheritance is closely tied to polymorphism, another key OOP principle. By allowing objects of different classes to be treated as instances of a common superclass, inheritance promotes flexibility and adaptability. For example, a "Vehicle" superclass can be used to represent both "Car" and "Bike" objects, enabling polymorphic behavior in methods like "drive."
Common Misconceptions About Inheritance
Despite its advantages, inheritance is often misunderstood. Some developers believe that inheritance is the only way to achieve code reuse, while others overuse it, leading to overly complex hierarchies. Below, we address these misconceptions and provide clarity on when and how to use inheritance effectively.
Inheritance vs. Composition
One common misconception is that inheritance is always preferable to composition. While inheritance is useful for modeling "is-a" relationships, composition is better suited for "has-a" relationships. For example, a "Car" class might use composition to include an "Engine" object rather than inheriting from it. Understanding the distinction between these approaches is crucial for designing clean and efficient code.
Avoiding Overuse of Inheritance
Overusing inheritance can lead to deep and convoluted hierarchies that are difficult to maintain. Developers should strive for a balance, using inheritance only when it genuinely simplifies the design. In many cases, composition or interfaces provide more flexible alternatives.
Real-World Applications of Inheritance
Inheritance finds applications in various domains, from gaming to enterprise software. For example, in gaming, inheritance is used to define a hierarchy of characters, each with unique abilities. Similarly, in banking software, inheritance helps model different types of accounts, such as savings and checking accounts.
What Are the Challenges and Limitations of Inheritance?
While inheritance offers numerous benefits, it also presents challenges. Overusing inheritance can lead to tightly coupled code, making it difficult to modify or extend. Additionally, multiple inheritance can introduce ambiguity, requiring careful design to avoid conflicts.
Frequently Asked Questions About Synopsis of Inheritance
What is the difference between inheritance and polymorphism?
Inheritance allows one class to inherit properties and methods from another, while polymorphism enables objects of different classes to be treated as instances of a common superclass. Both concepts are integral to OOP but serve different purposes.
Can inheritance be used in functional programming?
Inheritance is primarily associated with OOP. However, functional programming languages like Scala support similar concepts through traits and mixins, offering a blend of OOP and functional paradigms.
How can I avoid overusing inheritance?
To avoid overusing inheritance, consider using composition or interfaces where appropriate. Focus on designing shallow hierarchies and prioritize simplicity over complexity.
In conclusion, understanding the synopsis of inheritance is essential for anyone working with object-oriented programming. By mastering its principles and applications, developers can build efficient, scalable, and maintainable
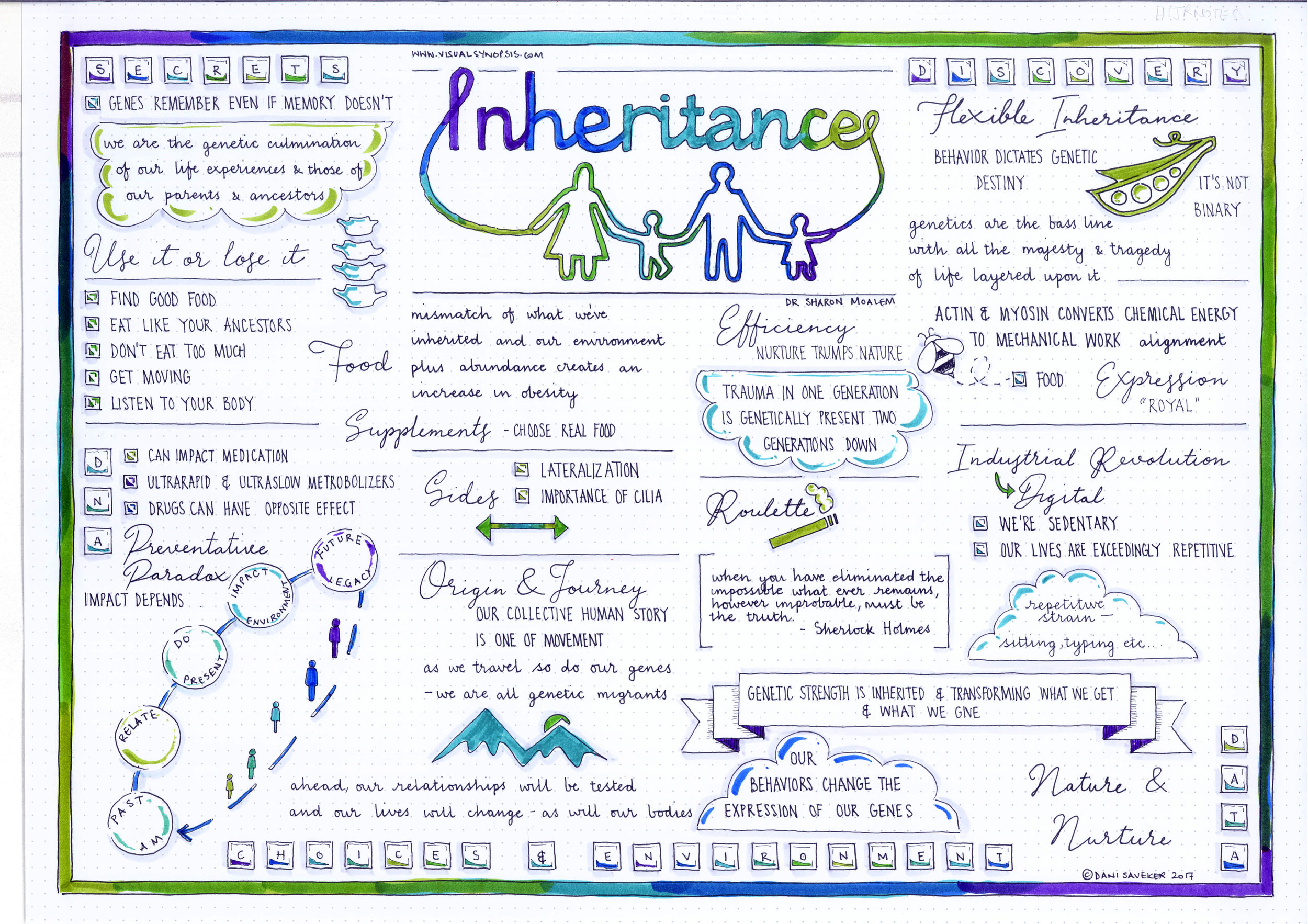
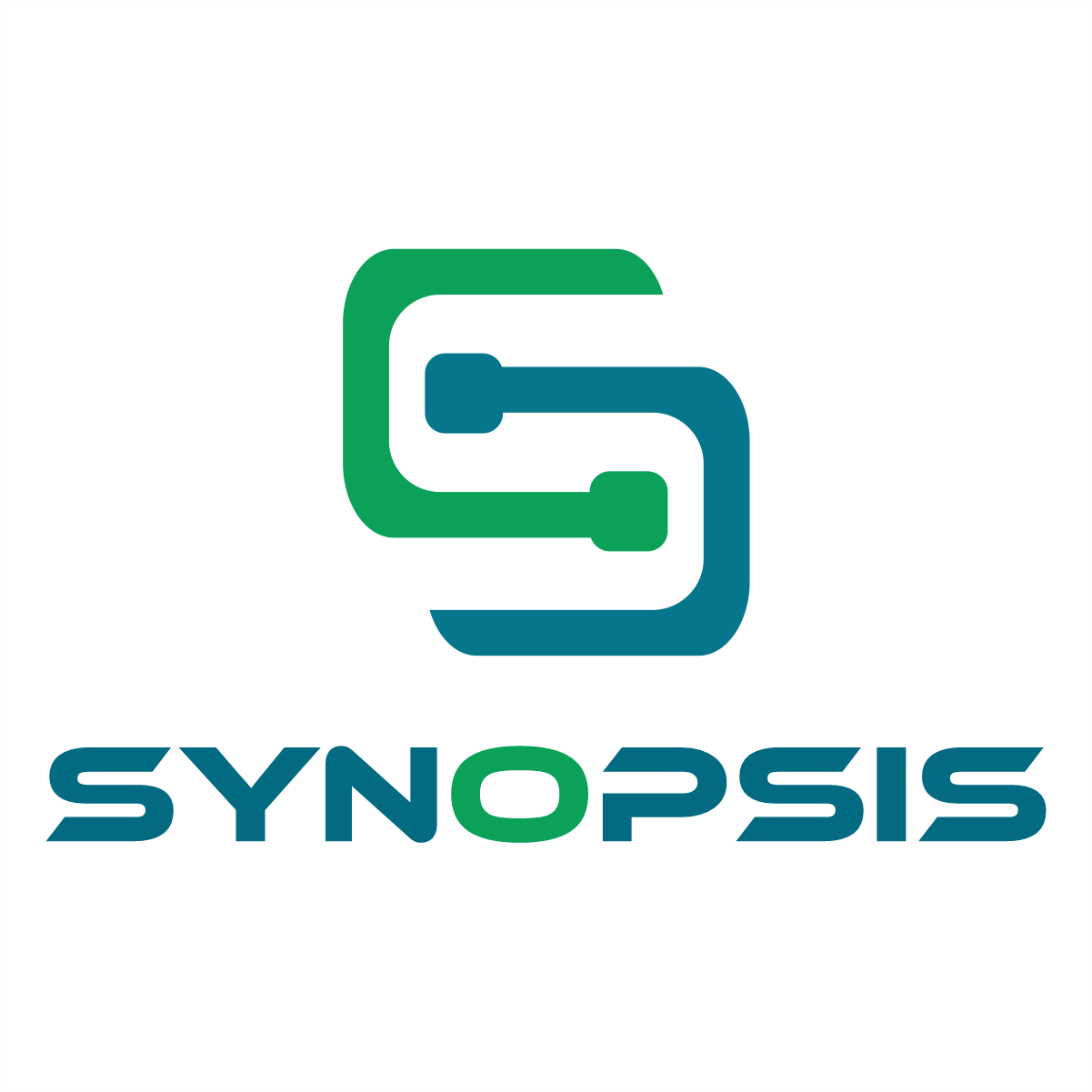